# Overview
Dedicated mobile (iOS & Android) applications are known for better client engagement and increased customer lifetime value (CLV), but, due to the complex review process of App Store and Google Play, as well as the time it takes for all clients to update to the latest version, it might be hard to continuously change and optimize the best flow for client verification and two-factor authentication.
To help fast-moving companies overcome these obstacles, CheckMobi delivers a feature called Remote Config
– an API-based capability that enables changing the mobile number verification, two-factor authentication, and one-time password flows without publishing an app update, at no extra cost and with unlimited changes per account.
Remote Config
works with our SMS, Voice, and Missed Call products. Now you can easily choose, directly from the CheckMobi web-console, which of these services will be your primary method for sending one-time passwords, whether to use a backup (giving your customers more options) or, how many times a client can retry sending a verification code.
All this with the flexibility of choosing different flows based on the platform (iOS or Android), country or mobile network operator.
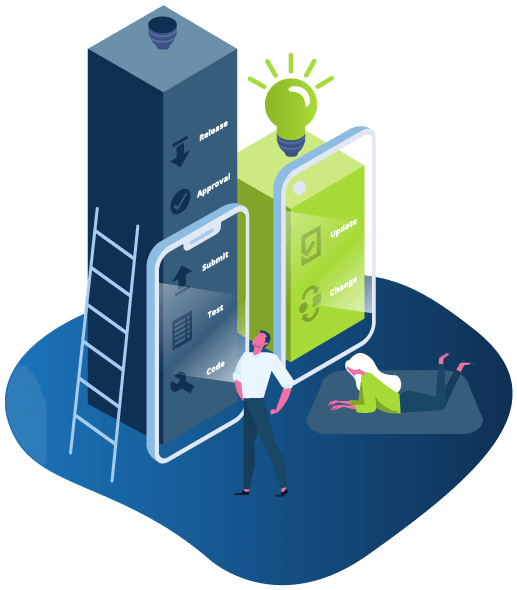
# What can I do with Remote Config?
App developers can use Remote Config in many different ways to speed up their development process. Here some real-life scenarios:
# Country-specific configuration to increase the success rate and reduce cost.
Countries differ greatly in which method works best for sending one-time passwords or how much it costs to send it. You could have countries where the cost of sending an SMS is a few times cheaper than sending a call while on others they can be similar. A client can use CheckMobi reports and analytics to monitor the performance of each country and then make changes on a country basis in the verification flow to reduce cost and increase the success rate.
# A/B Test multiple flows on the same destination.
Imagine you want to start improving the quality and reduce the cost for a top destination or mobile network where you have lots of customers. Let’s take for example France – Orange
network. You can choose a number prefix belonging to Orange
such as +33 413
, where you have active clients that request one-time passwords. Using Remote Config
you can easily create a flow for numbers starting with +33 413x
(where x is between 1,2,3,4,5) and another different flow for numbers starting with +33 413y
(where y is between 6,7,8,9,0). After enough clients have gone through both flows you can compare results and decide which flow is the winner when sending one-time passwords on France – Orange
mobile network.
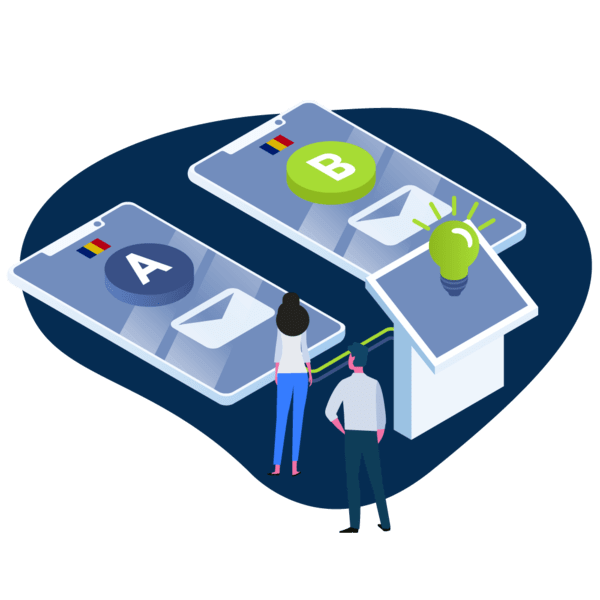
# Act fast on unexpected issues.
International SMS & Voice calling are complex services that stretch along many routes and network operators to deliver a world-wide product. But these complex networks can sometimes have temporary outages. Other times, due to country regulations the cost for SMS or for voice might suddenly increase. For all these unexpected cases, where you need to act fast and change the two-factor authentication flow, Remote Config can help a lot. You can easily do all these changes without going through the lengthy review process of App Store
and Google Play
and deliver the change instantly to all your app clients instead of waiting for them to update to the latest version.
# How to integrate it in my apps?
In order to integrate the Remote Config SDK into your app you need to follow the integration steps found on our Github repositories:
Hereinafter you can find how easy is to do a basic integration. For more details please check the Github Repositories above.
# Android
// In order to use the SDK you need to set the CheckMobi Secret Key from the web portal.
// You can do this somewhere before calling any SDK method by calling:
CheckmobiSdk.getInstance().setApiKey("YOUR_SERET_KEY_HERE");
// The first thing you need to do before starting the verification process is to
// check if the user has already verified his number. You can do this like so:
if(CheckmobiSdk.getInstance().getVerifiedNumber(<context>) != null)
{
// user already verified the number ...
}
else
{
// start a validation process you should add the following lines of code:
startActivityForResult(
CheckmobiSdk.getInstance()
.createVerificationIntentBuilder()
.build(StartActivity.this), VERIFICATION_RC);
}
// You should also override the onActivityResult method like so:
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent data) {
super.onActivityResult(requestCode, resultCode, data);
if (requestCode == VERIFICATION_RC) {
if (resultCode == RESULT_OK) {
//The user has verified his phone number successfully
} else {
//The user canceled the verification process
}
}
}
# iOS using Swift
// In order to use the SDK you need to set the CheckMobi Secret Key from the web portal.
// You can do this somewhere before calling any SDK method by calling:
CheckMobiManager.shared.apiKey = "YOUR_SERET_KEY_HERE"
// The first thing you need to do before starting the verification process is to
// check if the user has already verified his number. You can do this like so:
if(CheckMobiManager.shared.verifiedPhoneNumber != nil)
{
//user already verified the number ...
}
else
{
// start the verification process
CheckMobiManager.shared.startValidationFrom(viewController: self, delegate: self)
}
// You should also implement the CheckMobiManagerProtocol methods like so:
public func checkMobiManagerDidValidate(phoneNumber: String, requestId: String) {}
public func checkMobiManagerUserDidDismiss() {}
# iOS using Objective-C
// In order to use the SDK you need to set the CheckMobi Secret Key from the web portal.
// You can do this somewhere before calling any SDK method by calling:
CheckMobiManager.shared.apiKey = @"YOUR_SERET_KEY_HERE";
// The first thing you need to do before starting the verification process is to
// check if the user has already verified his number. You can do this like so:
if(CheckMobiManager.shared.verifiedPhoneNumber != nil)
{
//user already verified the number ...
}
else
{
// start the verification process
[CheckMobiManager.shared startValidationFromViewController:self delegate:self];
}
// You should also implement the CheckMobiManagerProtocol methods like so:
- (void)checkMobiManagerDidValidateWithPhoneNumber: (NSString *)phoneNumber
requestId:(String *)requestId {}
- (void)checkMobiManagerUserDidDismiss {}
After integrating this functionality into your apps, you can easily find the config capabilities in on the CheckMobi website by going in your Dashboard, under Settings -> [Your App Name] -> Remote Config SDK tab.
# Conclusion
If you are building mobile apps and want to move fast and deliver the best possible two-factor authentication flow at the lowest cost possible on every country that your product is available then CheckMobi's Remote Config feature is something you should consider implementing.